I have been learning PHP as my third programming language for a little while now. I started with the generic book studying and followed up with writing my own programs. As anyone who’s learnt or read about coding in PHP will know, everyone always ends up beginning with SQL and databases. I did the same. I created the database, accessed it and opened a connection. This is where I then moved on to the sign up and login system. In this article I will run through how I programmed the login portal and the methods I used to overcome the problems I had.
Database
This login portal is actually rather simple. Only needing one table for the users that sign up. The PHP code will access this table when a user signs up to insert their data and also to read the information and display it when someone logs in.Note: You should know that I named my database ‘php_login’. It’s not essential that you use this name but you should think about using a memorable name for your database connection.
I have used phpMyAdmin as the database program whilst building this. On the image below you will see the structure of my table. If you haven’t seen this layout before, it’s very easy to understand. The bold on the left is the name of column, the golden key means that field is the primary key. The grey keys are other fields that could be used as a primary key as they always need to be unique.
The Type is the next section. It’s quite easy to understand what to use for each one. Fields with just numbers will be ‘int()’, anything like a username or email that can contain letters or numbers will be ‘varchar()’. There are others such as ‘char()’ and ‘datetime’ that can be used but these are the only ones needed for the login system. The numbers inside the brackets represent the amount of characters that can be used in each field. The collation is a bit of a complication option and I will explain later why choosing ‘utf8_general_ci’ early on is a good idea.
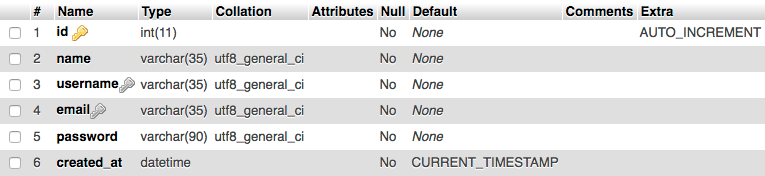
The first column I included was an ID. For nearly every database table you will create, it’s likely that you’ll need to include an ID column. This will always start at zero, there will never be a duplicate number and every record will increase the id number by one. Another good reason to have an ID column is to assign it as the primary key. A primary key is a unique field that will identify every single record in a database table. The ID isn’t always used as the primary key as there are other fields that always have to be unique such as a username, account number or product code.
I have only used the ID as the primary key field in this table as it is just the simplest column and best to identify.
The name, username and email fields are all relatively similar in that they are all varchar’s and I have decided to allow 35 characters in each field. 35 may be a little high but I thought it would be easier having the fields allow a few extra characters than having someone call me up saying their email address won’t work. Apart from the Collation option that I have said I will talk about later, this is all I have changed for these fields.
Next is the password. Obviously I used varchar again here because almost everyone tries to get a couple of numbers and symbols in their passwords. The 90 character limit is the result of a two day long fight I had with my PHP program. I will talk more about this later but to put it simply, the program uses the password_hash() function for encryption and this results in a string of at most 72 characters long. 90 is used just as a precaution.
The created_at field is another that isn’t essential but can be very useful. It records the immediate time and date that the user signed up.
The Pages
For the most basic php login and sign up form you will need five pages. I have used six since I added a logout function upon completing the project. You’ll need to start with a database connection file.db_connect.php
I named my database connection file ‘db_connect.php’. This file isn’t essential, since you could just include the few lines of PHP code you need for the connection at the top of every connection page. At the start of this file you need to declare some variables. These variables are used for information about the database. You can see on the code below that we start with the servername.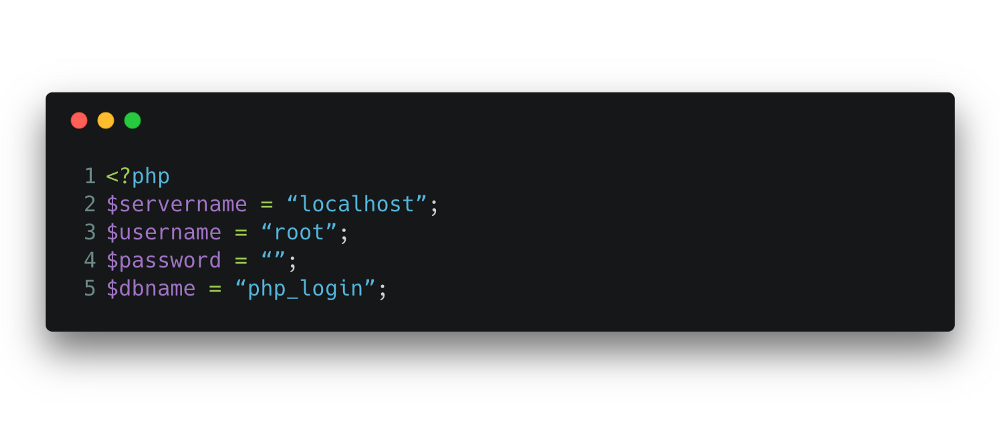
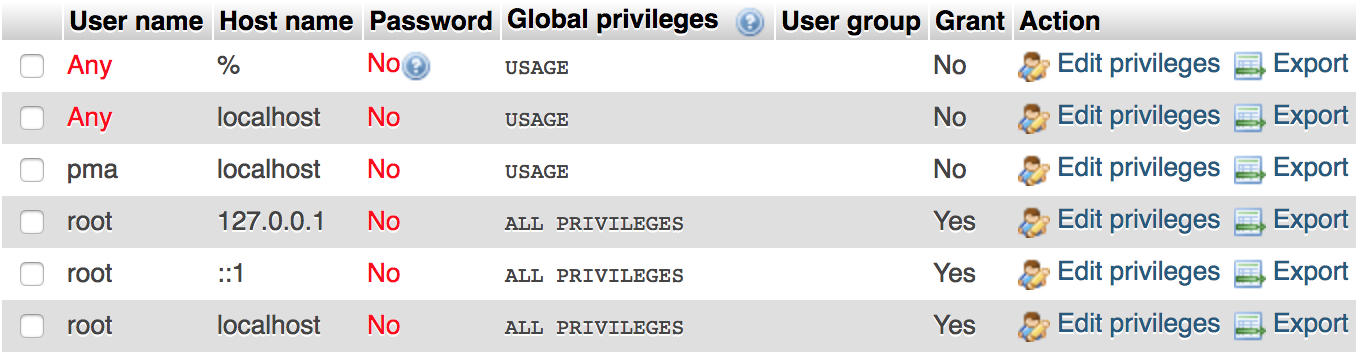
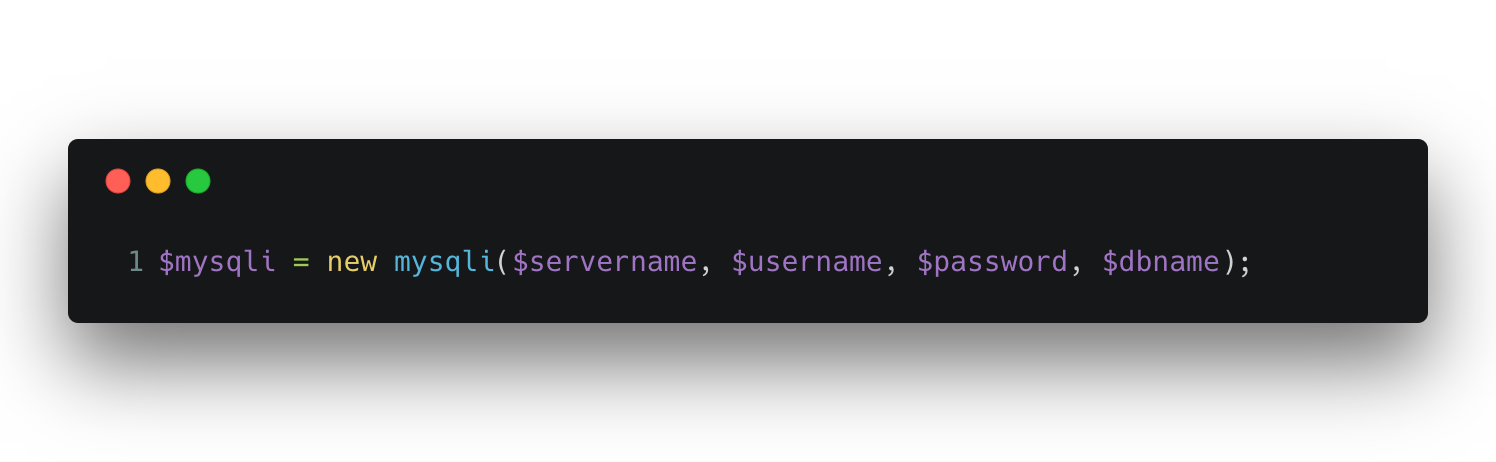
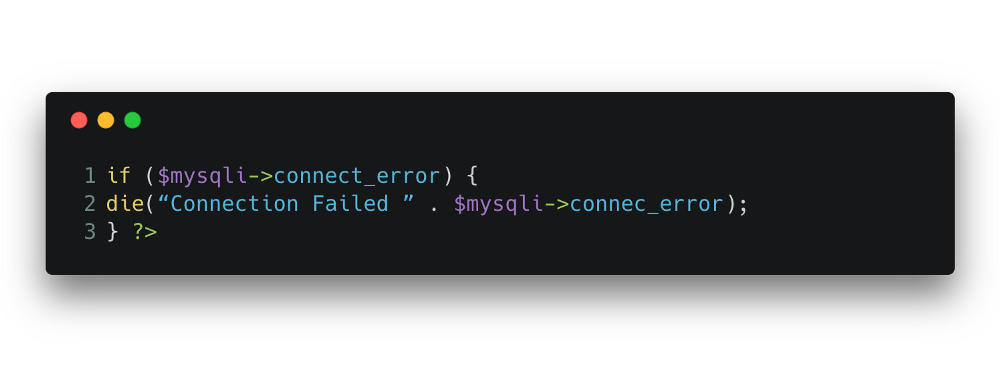
index.php
The next page that needs to be created is the index page. This is the first page any user will see so it needs to be aesthetic and make people want to use it. As for the images, introduction and anything else on this page, it doesn’t matter as long as you have a login and signup form somewhere. For this stage of the project, I only added these two forms as it would’ve wasted too much time to make it look pretty if customers weren’t going to use it.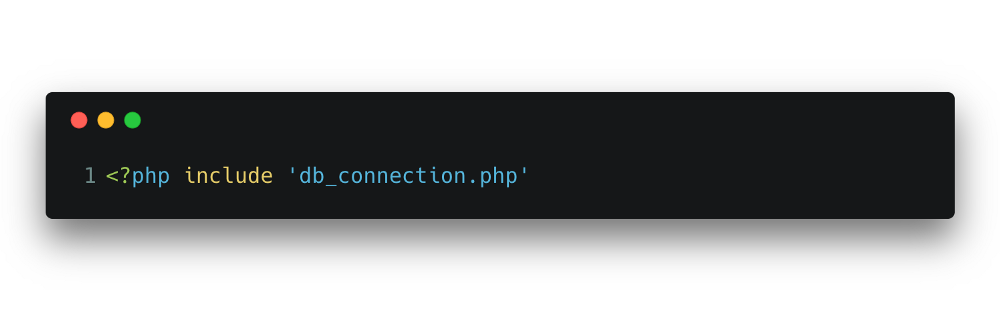
Now I’m connected to the database I can open my HTML tags and start shaping the page. The HTML tags are as simple as I can make them: html, head, charset, title and body tags to begin with. As you can see from the code below, I have started with the signup form.
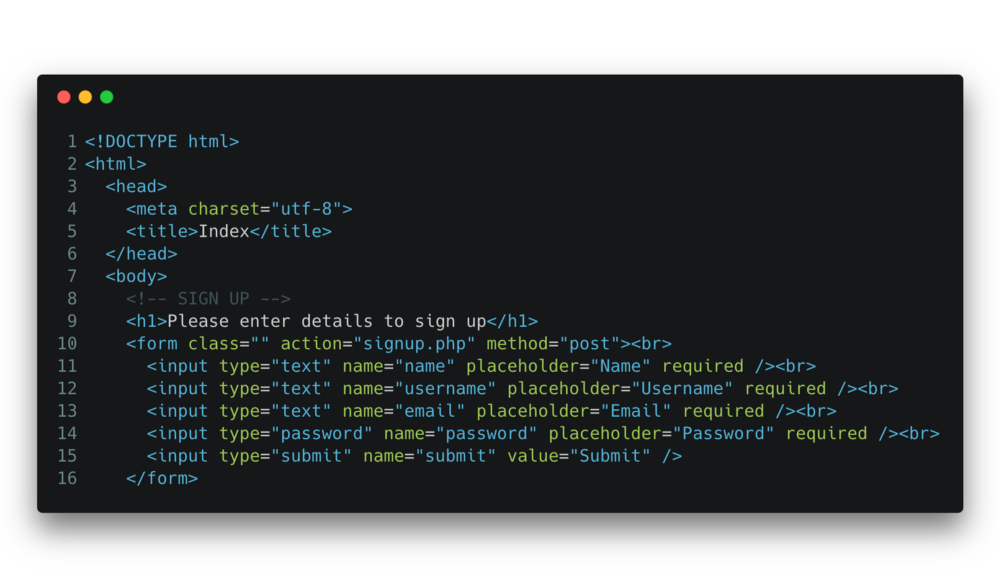
Next we get to the input tags. Since my form is very simple I only needed four input boxes and one submit tag. If it’s not evident, you need one input tag for each information section on your database table. The type attribute for the name, username and email can be ‘text’ because there is no data to be hidden and all the form is collecting is text. The password tag needs the ‘password’ type as what the user writes needs to be protected. The name attribute is simply giving a name to the tag. This name will be used to reference given to it when we get to the PHP. The placeholder text is not imperative. Placeholder text is what is written in the box before the user writes in it. As soon as the box is clicked this text will disappear and allow the user to write what they want. It is simply used to make forms easier to understand and use. Finally there’s the submit button. This can be done in a variety of ways but since I am making this project as simple as possible I will just use another input tag. Both the type and name attributes will be ‘submit’ because HTML already provides styling for submit buttons. For the writing inside the button you need to use a ‘value’ tag because the writing obviously needs to stay there when the button is clicked.
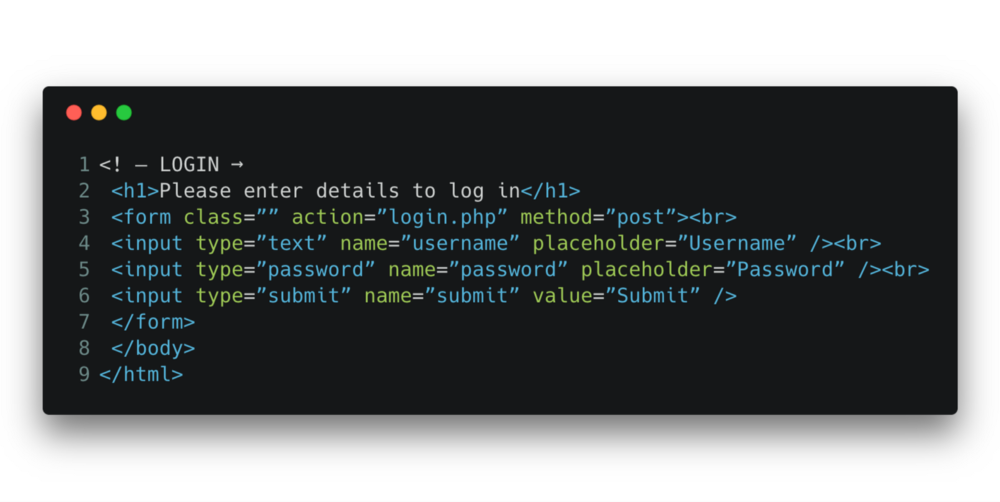
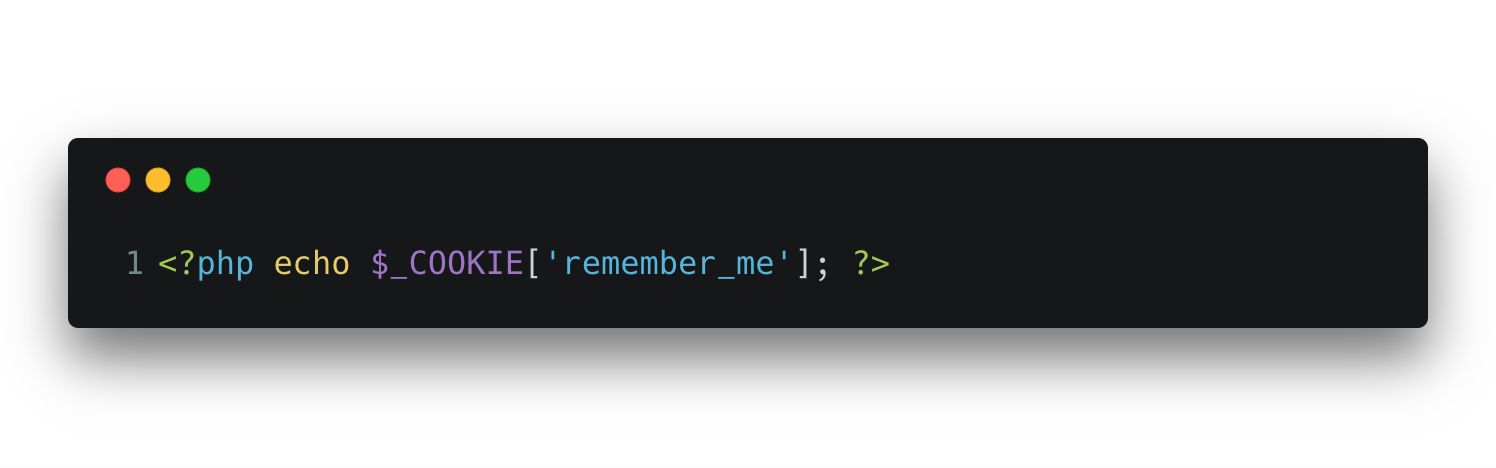
Login and Sign Up Form
signup.php
These two files are where I did most of the PHP coding. When you look at it now it’s actually quite simple code but when I was first writing it there are a few things I just could not get my head around. Firstly i’m going to walk through the signup page.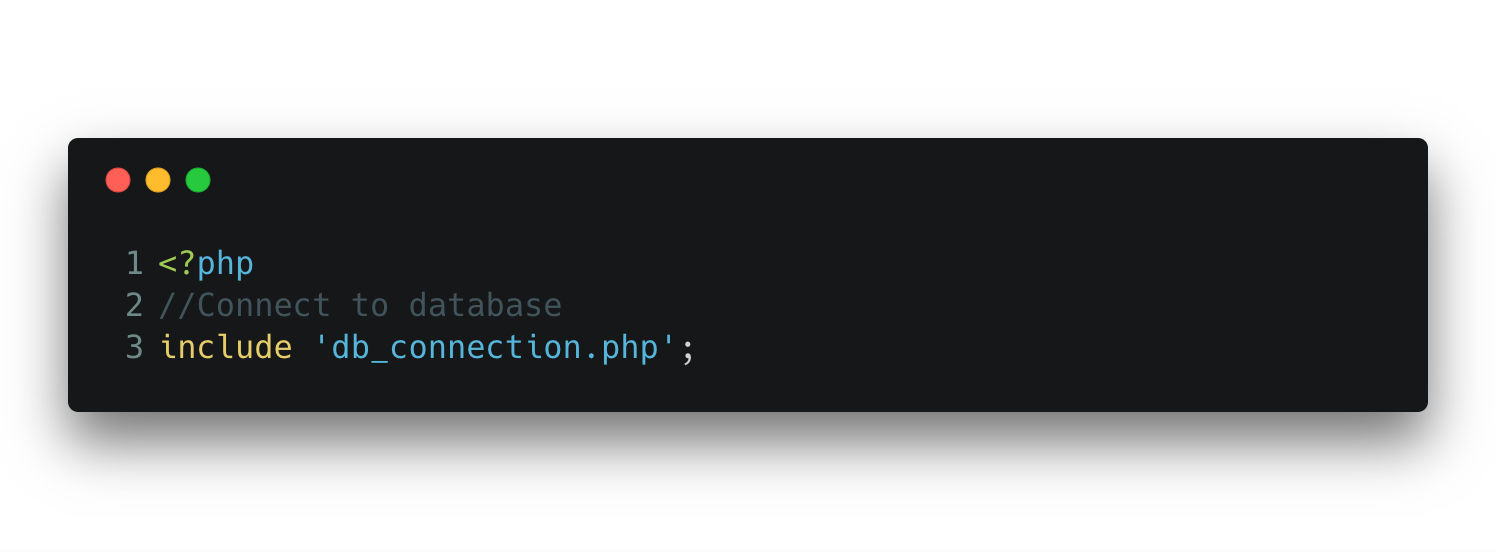
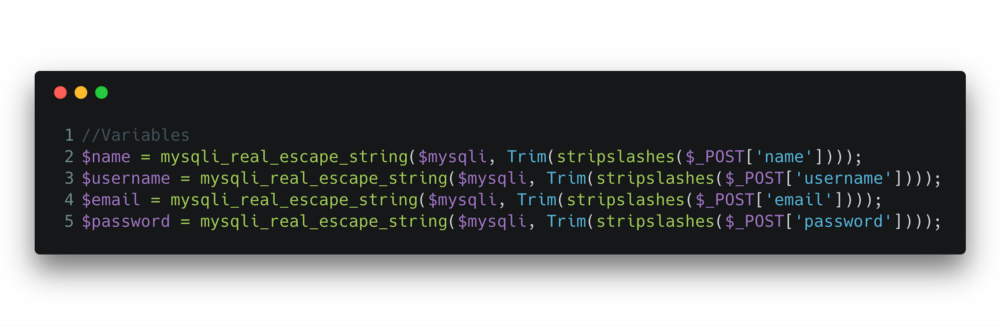
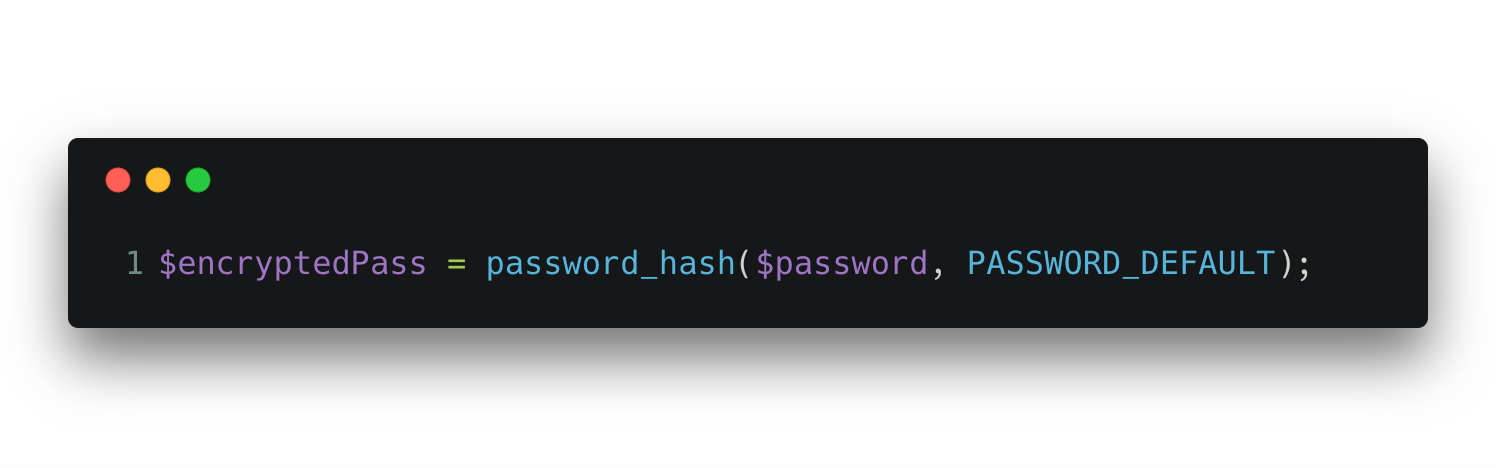
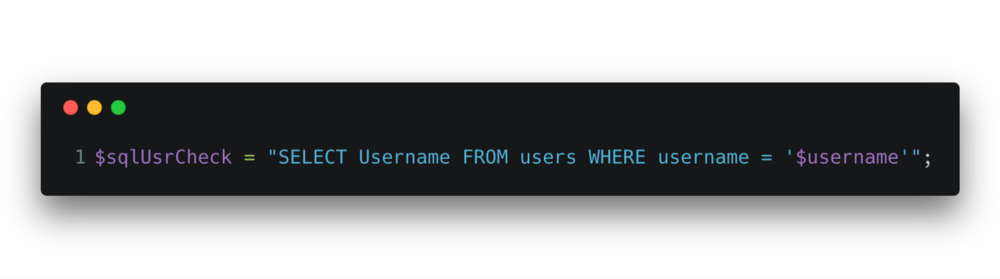
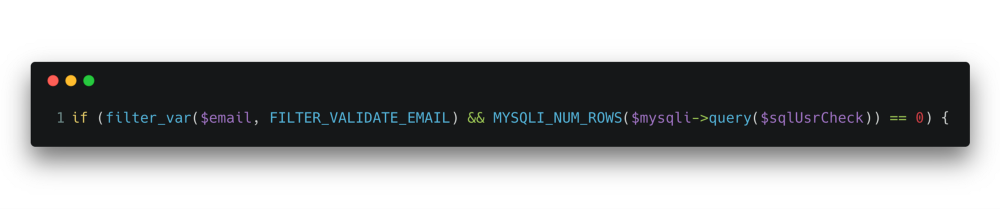
Essentially this if statement will let the user get to the next part of the code if the email is valid and if there are no listings output from the sql query (the username check).
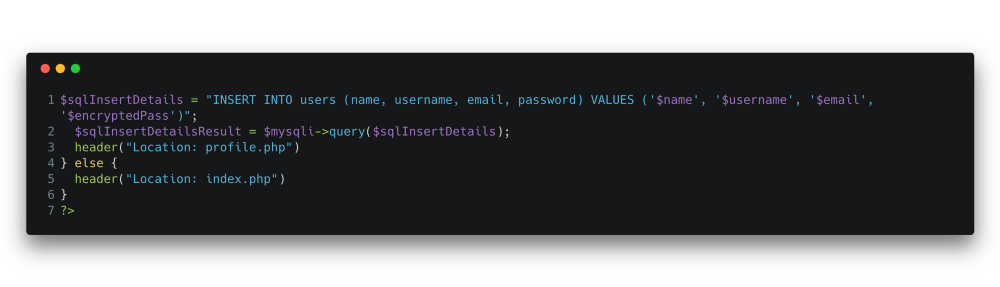
login.php
The next page is the login PHP page. Instead of checking details and then inserting them into the database, this page will check the login information and if it’s correct the user will go through to the profile page and if it’s not they will stay on the index page.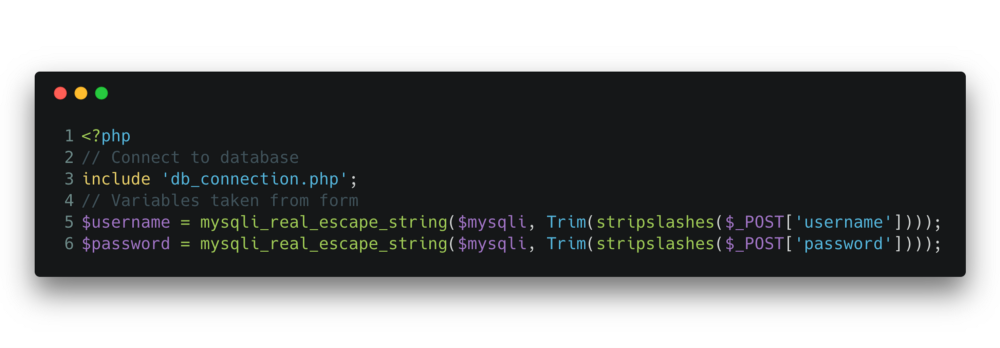
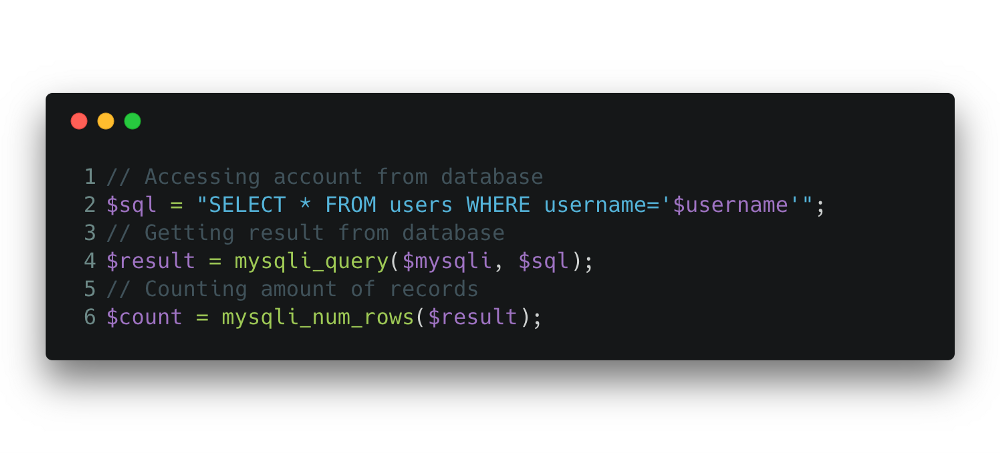
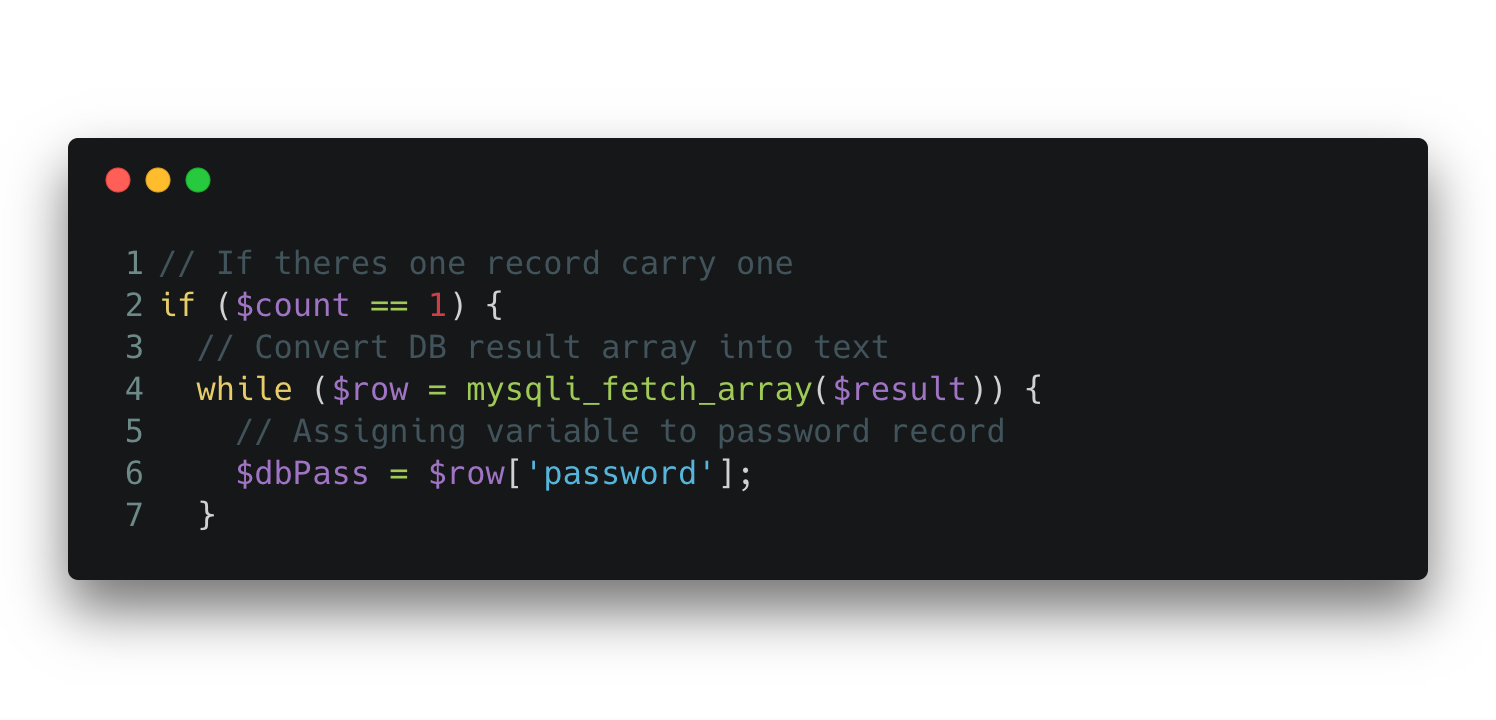
The code inside the if statement checks the password. When you collect data from a database it is in the form of an object, we need it to be a string to be able to verify the password. To do this we start with a while loop that will go through each record output from the database (which will only be 1) and it will assign the array to the variable $row. We will then go inside the while loop and change the row we get from the SQL query from the username column to the password column and assign it to the $dbPass variable. Now we have the encrypted password as a string.
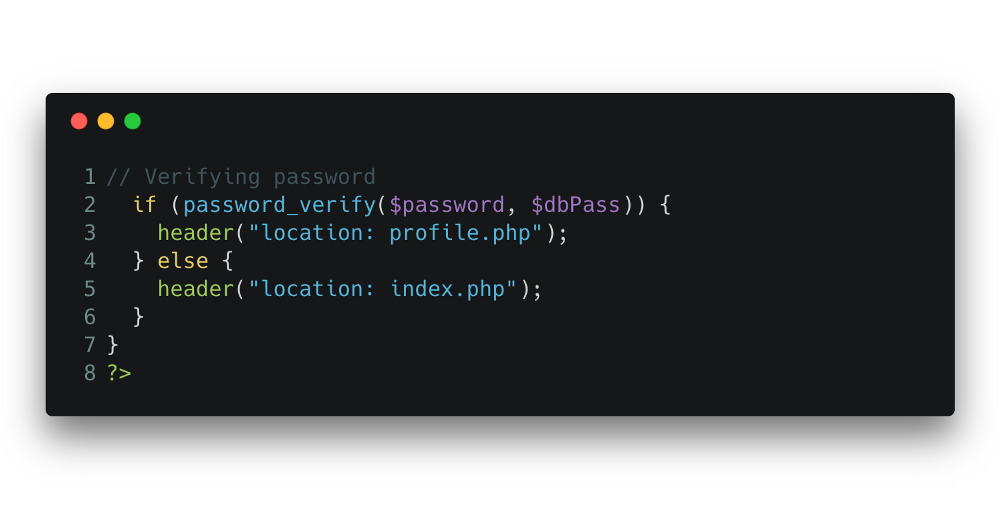
Now we have the encrypted password as a string we can use the password_verify() function to check it. The only way to check if something matches the password_hash() function. There is no other way of decryption. To use this function we need to give the user given password and the database given password. The function then does its magic and checks them against each other and since this is inside an if statement, the function will let us through if the passwords match. As you can see if they do we will go to the profile.php page and if not we will stay on index.php.
profile.php
The profile.php page is the page you go to after a successful signup or login. The actual code on this page doesn’t matter much for this project. You can simply say “Welcome” or you could initialise some session variables and personalise the welcome page. Right now I only have a welcome message on this page. You can see the code below.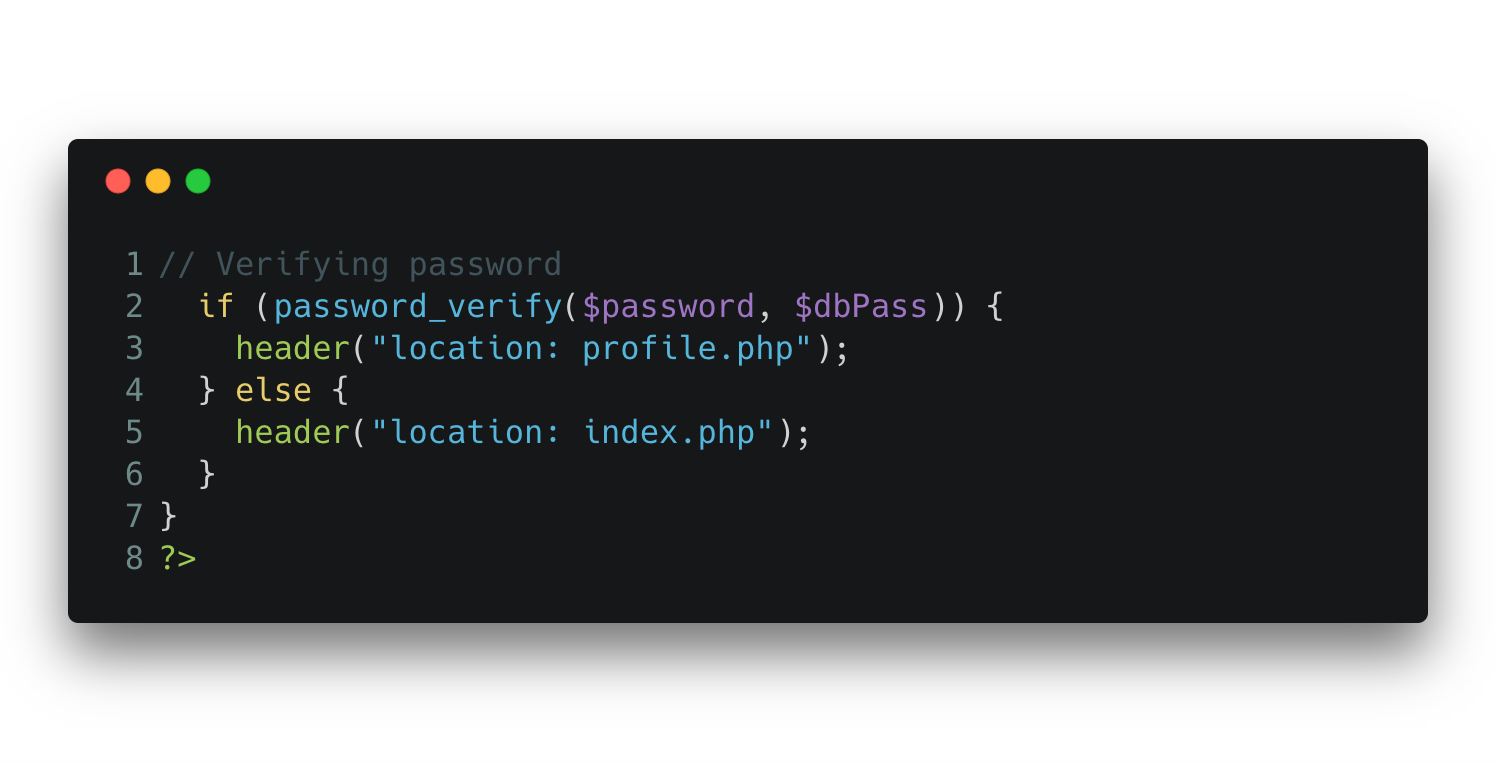
Conclusion
The code I have provided above will give you one of the most basic login forms possible. Unfortunately this means it isn’t the most secure login portal you could make. This code is perfectly good to use for your project but if you are storing a large amount of sensitive customer information you should definitely look to implement some better security measures.Be the first to comment...
0 Comments